JavaScriptのfocus
とblur
イベントは、フォーム要素やインタラクティブなUI要素でのフォーカス操作に関連するイベントです。これらを活用することで、入力フィールドの強調表示やエラーメッセージの表示など、より直感的でインタラクティブなインターフェースを構築できます。
目次 非表示
- 要素がフォーカスされたときに発生します。
- 通常、ユーザーがマウスでクリックしたり、タブキーを使用して要素にフォーカスを移動したときにトリガーされます。
- 要素がフォーカスを失ったときに発生します。
- 例えば、ユーザーが別の要素に移動したり、ブラウザの外をクリックした場合などにトリガーされます。
focus
とblur
イベントはバブリングしませんが、キャプチャフェーズで検知可能です。
以下は、focus
とblur
イベントを使った簡単な例です。
See the Pen focus() / blur() by tones (@tonescodedesign) on CodePen.
const input = document.getElementById('name');
const errorMessage = document.getElementById('error-message');
// フォーカス時に強調表示
input.addEventListener('focus', () => {
input.style.border = '3px solid green';
errorMessage.style.display = 'none';
});
// フォーカスを失ったときにバリデーション
input.addEventListener('blur', () => {
if (input.value.trim() === '') {
errorMessage.style.display = 'block';
}
});
<form>
<label for="name">名前:</label>
<input type="text" id="name">
<p id="error-message" class="error" style="display: none;">名前を入力してください。</p>
</form>
input:focus {
outline: 2px solid blue;
}
.error {
color: red;
font-size: 0.9em;
}
フォームの入力値をリアルタイムでバリデートする例です。
See the Pen focus() / blur() by tones (@tonescodedesign) on CodePen.
const emailInput = document.getElementById('email');
const validationMessage = document.getElementById('validation-message');
emailInput.addEventListener('blur', () => {
const email = emailInput.value;
if (!email.includes('@') || !email.includes('.')) {
emailInput.classList.add('invalid');
emailInput.classList.remove('valid');
validationMessage.style.display = 'block';
} else {
emailInput.classList.add('valid');
emailInput.classList.remove('invalid');
validationMessage.style.display = 'none';
}
});
emailInput.addEventListener('focus', () => {
validationMessage.style.display = 'none';
});
<label for="email">メールアドレス:</label>
<input type="email" id="email">
<p id="validation-message" style="color: red; display: none;">無効なメールアドレスです。</p>
.valid {
border-color: green;
}
.invalid {
border-color: red;
}
focus
イベントを使って、現在フォーカスしているフィールドを強調表示します。
See the Pen focus() / blur() by tones (@tonescodedesign) on CodePen.
const inputs = document.querySelectorAll('input');
inputs.forEach(input => {
input.addEventListener('focus', () => {
input.classList.add('highlight');
});
input.addEventListener('blur', () => {
input.classList.remove('highlight');
});
});
<form>
<label for="username">ユーザー名:</label>
<input type="text" id="username">
<br><br>
<label for="password">パスワード:</label>
<input type="password" id="password">
</form>
.highlight {
background-color: lightyellow;
}
focus
とblur
は他のイベントとは異なり、親要素に伝播しません。そのため、フォーム全体に対してイベントを検知したい場合は、focusin
やfocusout
を使用する必要があります。
document.addEventListener('focusin', (event) => {
console.log(`${event.target.tagName}がフォーカスされました。`);
});
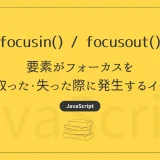
複数の入力欄を含むフォームでは、個々のfocus
やblur
イベントにリスナーを追加するよりも、focusin
やfocusout
を活用する方が効率的です。
フォーカス時に表示される内容が視覚的なものに限定される場合、スクリーンリーダー利用者には認識されない可能性があります。必要に応じてaria-live
属性や適切な説明文を追加しましょう。
<div id="help" aria-live="polite">フォーカスされたときの説明をここに表示します。</div>